Let’s discuss regarding convert Javascript JSON ( Javascript Object Notation ) to string or javascript JSON to string.
We are using JSON.stringify() for JSON to string converter.
Let’s check with an example:
let json_string = JSON.stringify({ Class: 1, Subject: 6 }) console.log(json_string); //Output: "{"Class":1,"Subject":6}"
In the above example :
let used for declaring the variable in javascript.
JSON.stringify() method converts a JavaScript value into a JSON string.
console.log() method print value in the console of the browser.
let’s convert a JavaScript array to JSON:
var prog_lang = ['javascript', 'ruby', 'java', 'c']; var json = JSON.stringify(prog_lang); // '["javascript","ruby","java","c"]'
JSON.stringify with Unsupported Data Types
Supported JSON Data types:
- Strings - numbers - booleans - null - arrays - objects
Un – supported JSON Data types:
- Date objects - regular expressions - undefined - functions
Let’s Check with Example:
var json_unsupported = { name: 'Test', no: 10, date: new Date(), code: ['c', 'c++', 'java'], object: { no: 10, like: true, name: null, value: undefined, exp: /^\d+$/, fun: function(value) { // code here } } } var json_string = JSON.stringify(json_unsupported); console.log(json_string); /* {"name":"Test","no":10,"date":"2018-10-28T03:07:00.520z", "code":["c,"c++","java"], "object":{"no":10,"like":true,"name":null,"exp":{}}} */
In the above example:
var used for declaring the variable in javascript.
JSON.stringify() method converts a JavaScript value into a JSON string.
console.log() method print value in the console of the browser.
exp (regular expression) – has been converted to an empty object.
name with undefined – has been removed.
fun (function) with values has been removed.
JSON.stringify with Indent or Space:
JSON.stringify’s output does not contain default white space. it compact for data transmission but difficult to read. So we can use its optional argument for space or indent.
var json_data = { name: 'Test', no: 10, date: new Date(), code: ['c', 'c++', 'java'], object: { no: 10, like: true, name: null } } var json = JSON.stringify(json_data, null, 4); console.log(json); /* output of console.log with indent of 4 { name: 'Test', no: 10, date: new Date(), code: ['c', 'c++', 'java'], object: { no: 10, like: true, name: null } }
In the above example, the third argument is 4 and its use for indent. The second argument was passed as null because we need the third argument for indent.
You can refer to https://www.learningcontainer.com/json-example/ for the JSON format example.
You can compare JSON file, data and URL on https://jsoncompare.org its best tool for comparing with details.
You can format your JSON file and URL and Data directly on https://jsonbeautifier.org – it is a very powerful and easy tool for view JSON data.
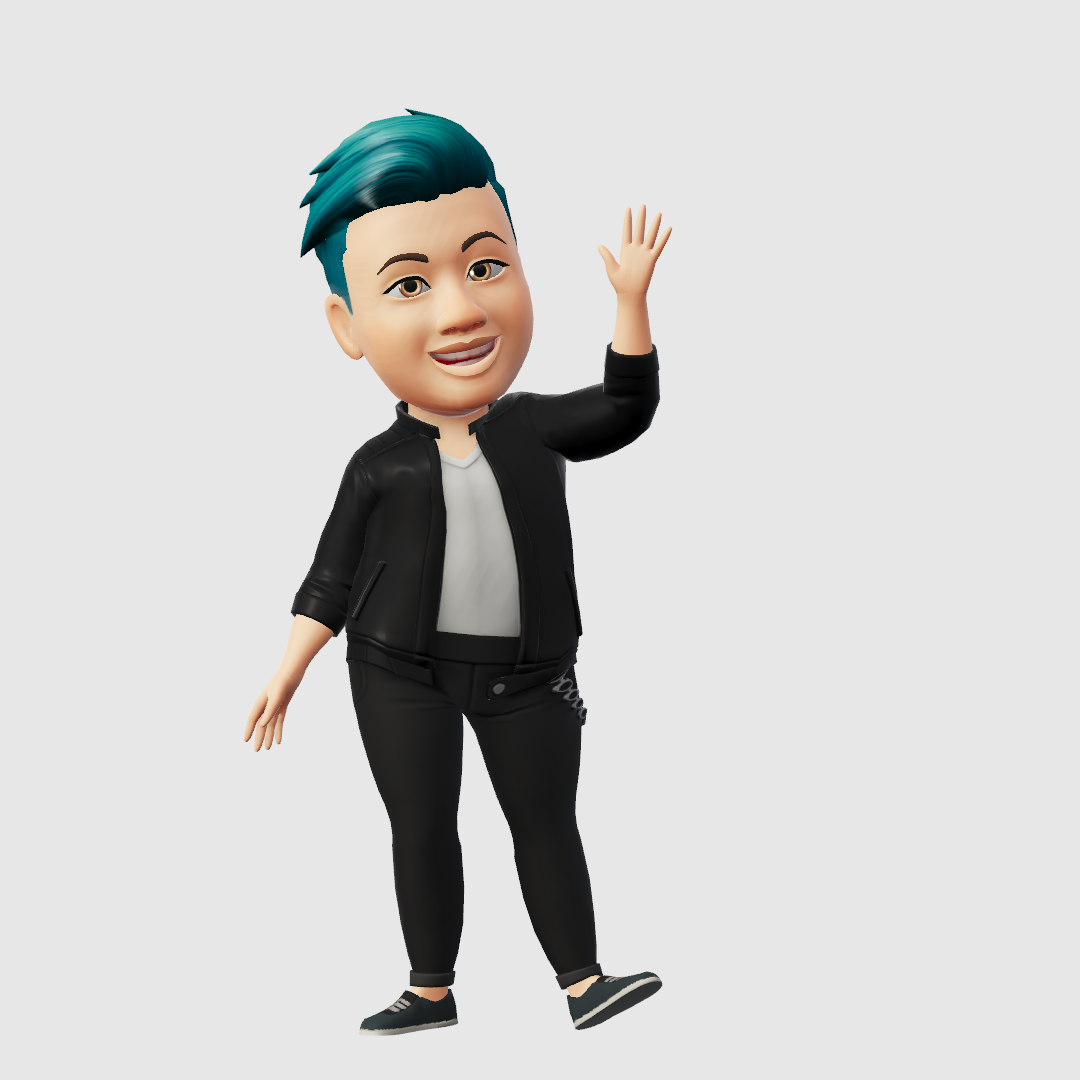
Hello, I am Denail Soovy. I am a developer of different technology. I am passionate about teaching and Daily teaching many students. I want to share knowledge with all of the developers or other people who need it.
I will try to teach every student with my easy and updated blogs.